Java programming examples: Create a useful application to generate random matches of doubles teams
In this article, I would like to show how real-life problems can be easily solved using the Java programming language.
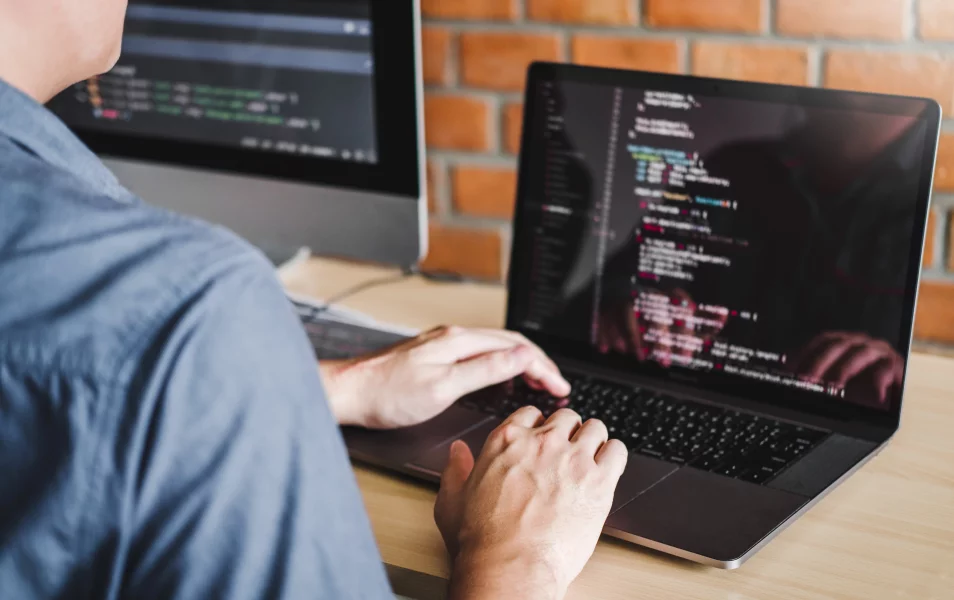
V článku sa dozvieš:
Assignment
Together we are going to write a program that will create unique pairs out of N registered individuals and randomly generate 2 teams of pairs to play against each other. The aim is to create all possible match variations so that each team can play against the remaining teams.
Suggested solution
If you are solving a more complex problem, it is advisable to break it down into smaller parts and solve them separately. In the case of this task, it could be divided into the following steps:
- retrieving the registered persons and storing them in the program memory,
- creating variations of pairs without repetition,
- creating all possible games for two pairs,
- randomly shuffling the generated games,
- listing the results.
We will now look at each step in more detail.
Retrieve the registered persons and store them in the program memory
In this section we read the list of logged in people from the file and store them in the people list. A person is represented by the Person class.
Create variations of pairs without repetition
When we have loaded people, we need to create all possible pairs of them, so that the people in them do not repeat, and of course each pair should be unique. In a pair, we don’t care about the order of who is the first or second member. Such a pair is represented by the class Pair, and the results are stored in the UniquePairs list.
Create all possible games for two pairs
From the two-person teams that have been formed, we will create as many games as possible for each team to play against each other. However, we need to ensure that a given pair can only play in one team in a game, i.e. pairs cannot play against each other in a game, and no player can play in two teams at the same time. A game is represented by the Match class, and we will store these in the Matches list for further processing.
Shuffle generated matches randomly
As an extension, we’ll add an element of randomness by randomly shuffling the generated matches.
List the results
For this type of console application, it will be sufficient to output the results to the console.
Design implementation in the Java programming language
Once we have identified the main steps of the program, we have no choice but to program it. We are going to create a tournament system for matches between groups of teams (pairs of people). To give you a better idea of what the program does, I’ll explain it step by step:
Person.java
package entities;
public class Osoba {
public String meno;
public Osoba(String meno) {
this.meno = meno;
}
}
It defines a Person class with a Name attribute representing the person’s name.
Pair.java
package entities;
public class Dvojica {
public Osoba osoba1;
public Osoba osoba2;
public Dvojica(Osoba osoba1, Osoba osoba2) {
this.osoba1 = osoba1;
this.osoba2 = osoba2;
}
}
It defines the Pair class, which contains two people (person1 and person2) and represents a pair of people or a two-man team.
Match.java
package entities;
public class Zapas {
public Dvojica tim1;
public Dvojica tim2;
public Zapas(Dvojica tim1, Dvojica tim2) {
this.tim1 = tim1;
this.tim2 = tim2;
}
}
The Match class represents a match between two pairs or teams (team1 and team2).
Generator.java
package entities;
import entities.*;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
public class Generator {
private List<Osoba> ludia;
private List<Dvojica> unikatneDvojice;
private List<Zapas> zapasy;
public void Generator() {
ludia = null;
unikatneDvojice = null;
zapasy = null;
}
public void nacitajVstupneData() throws IOException {
ludia = new ArrayList<>();
for(String line : Files.readAllLines(Paths.get("vstupy.txt"))) {
ludia.add(new Osoba(line));
}
}
// Vytvori všetky možné dvojice z ľudí
public void vygenerujUnikatneDvojice() {
unikatneDvojice = new ArrayList<>();
for (int i = 0; i < ludia.size(); i++) {
for (int j = i + 1; j < ludia.size(); j++) {
Dvojica dvojica = new Dvojica(ludia.get(i), ludia.get(j));
unikatneDvojice.add(dvojica);
}
}
}
// Vytvori všetky možné zápasy s danými dvojicami
public void vygenerujUnikatneZapasy() {
zapasy = new ArrayList();
for (int i = 0; i < unikatneDvojice.size(); i++) {
for (int j = i + 1; j < unikatneDvojice.size(); j++) {
Dvojica tim1 = unikatneDvojice.get(i);
Dvojica tim2 = unikatneDvojice.get(j);
if(tim1.osoba1.equals(tim2.osoba1) || tim1.osoba1.equals(tim2.osoba2) || tim1.osoba2.equals(tim2.osoba1) || tim1.osoba2.equals(tim2.osoba2))
continue;
zapasy.add(new Zapas(tim1, tim2));
}
}
}
public List<Osoba> getLudia() {
return ludia;
}
public List<Dvojica> getUnikatneDvojice() {
return unikatneDvojice;
}
public List<Zapas> getZapasy() {
return zapasy;
}
}
The Generator class is responsible for loading, processing the data and generating the results. It uses three lists (people, uniquePairs and matches) to manage data about people, teams and matches.
Generator() constructor – initializes the lists to null.
Method nacitajInputData() – reads data about people from the file inputs.txt and creates objects of Person type from it, which it stores in the People list.
GenerateUniqueDoubles() method – creates all possible pairs of people and stores them in the uniquePairs list.
GenerateUniqueMatches() method – creates all possible matches between pairs and stores them in the Matches list. It ensures that no two individuals are repeated in different pairs.
Access methods getPeople(), getUniquePairs(), getMatches() – provide access to individual lists.
Main.java
import java.io.IOException;
import java.util.Collections;
import java.util.Random;
import entities.*;
public class Main {
public static void main(String[] args) throws IOException {
Generator generator = new Generator();
generator.nacitajVstupneData();
System.out.println("Prihlaseni ludia [" + generator.getLudia().size() + "]: ");
for (Osoba osoba : generator.getLudia()) {
System.out.println(osoba.meno);
}
System.out.println();
generator.vygenerujUnikatneDvojice();
System.out.println("Mozne dvojice [" + generator.getUnikatneDvojice().size() + "]: ");
for (Dvojica dvojica : generator.getUnikatneDvojice()) {
System.out.println(dvojica.osoba1.meno + " a " + dvojica.osoba2.meno);
}
System.out.println();
generator.vygenerujUnikatneZapasy();
Collections.shuffle(generator.getZapasy(), new Random());
System.out.println("Zapasy kazdy s kazdym v nahodnom poradi [" + generator.getZapasy().size() + "]: ");
for (Zapas zapas : generator.getZapasy()) {
System.out.println(zapas.tim1.osoba1.meno + " a " + zapas.tim1.osoba2.meno + " vs. " +
zapas.tim2.osoba1.meno + " a " + zapas.tim2.osoba2.meno);
}
}
}
Main.java contains the main() method, which demonstrates the functionality of the programme. It creates an instance of the Generator class. It retrieves the input data about people and lists the logged in people. It generates all possible unique pairs and lists them. It generates all possible matches, then randomly sorts them and prints them to the console.
The program works for any number of people and can be easily modified and adapted to suit your own needs.
Program Input:
Program Output:
Exercise 1
Add the gender attribute (male, female) to the Person class. Modify the generateuniquepairs() method to generate all possible pairs of people, so that each team consists of a man and a woman. Then generate possible matches. Save the results in the output file.
Exercise 2 (challenge)
When creating a generator instance, specify a number that determines how many members the teams you are creating will have. Then modify the code in the example to work for teams with any number of members.
Java code example
We have prepared the files with the above example as code that you can run directly in Java. Please download the Java code example from here.
If you’re comfortable creating code from scratch and you’re an experienced Java developer, check out our employee benefits and respond to our job offers!