Java game coding tutorial for beginners: How to code a game?
Have you ever wanted to learn the Java programming language but didn’t want to slog through lengthy tutorials? What if you could build a game – your own application – while gradually mastering programming basics? Game programming might seem daunting for beginners, but this article will show you that creating your first app doesn’t have to be complicated. In fact, we promise it’ll be fun! Follow along as we build a number guessing game in Java, and you’ll see just how accessible coding can be.
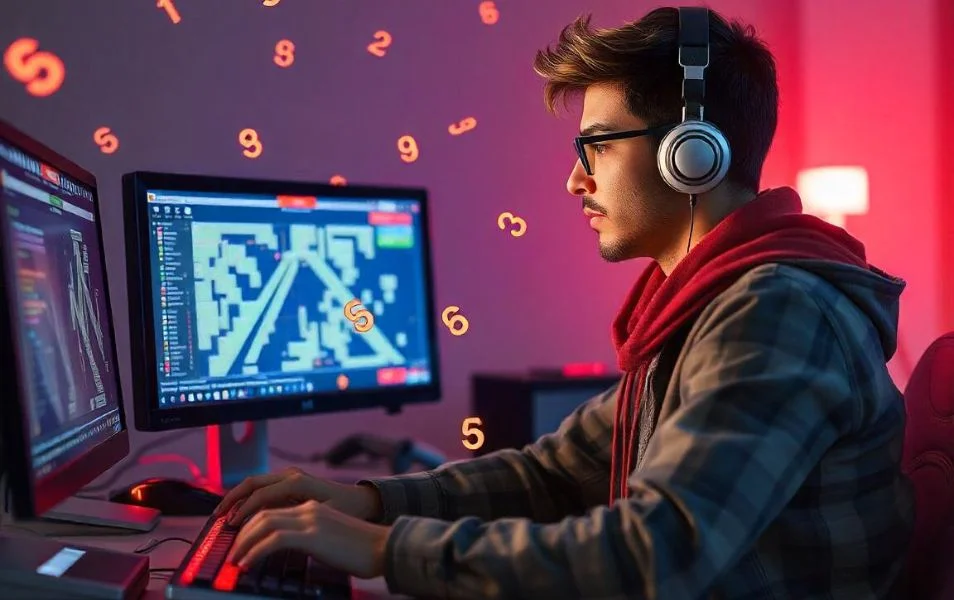
V článku sa dozvieš:
Let’s start with the Java game ‘Guess the Number’
Why a number guessing game? This Java game is an ideal exercise for beginners for several reasons. Simple games can be a great introduction to the world of coding because there is beauty in simplicity. The game is based on a simple concept – the player has to guess a randomly generated number within a given range. No complex algorithms or advanced techniques are required.
We will practice a combination of the basic principles of how to interact with the app user, how to elicit input from the user, and how to process the user’s answers.
You will learn how to work with:
- Data types – numbers, texts, variables
- Conditions (checking if the answer is correct)
- Cycles (repeated attempts by the user)
- Random number generation (which adds dynamism and randomness to the game)
This interesting Java game lets you understand how the different parts of a program come together to form a whole. Try programming this game – not only will you have fun, but you’ll learn a lot at the same time. Programming for beginners isn’t about creating big applications right away, it’s about the joy of solving small problems. And this game is a great start!
‘Guess the Number’ game rules
The game will give you several attempts, during which you will guess a number that the computer has randomly generated. After each guess, the game will tell you if you’ve got it right, or if you should try a smaller or larger number. If you guess correctly, the program will praise you. If not, it will tell you the correct number after all attempts have been made.
Ready to dive into the world of Java and create your own game?
Java game implementation
This program is a simple number guessing game in Java. The player has to guess a randomly generated number from a certain range in a limited number of attempts.
NumberGuessing Class
package games;
import java.util.Scanner;
public class NumberGuessing {
private int min, max, attempts, guess;
private final String
text1A = "Hadaj cislo medzi ",
text1B = " a ",
text2A = "Mas ",
text2B = " pokusov.",
text3 = "Hadaj cislo:",
text4 = "Cislo je vacsie ako ",
text5 = "Cislo je mensie ako ",
text6 = "Smola, neuhadol si. Cislo bolo ",
text7 = "Vyborne! Uhadol si cislo.";
public void createGame(int min, int max, int attempts) {
this.min = min;
this.max = max;
this.attempts = attempts;
}
public void play()
{
Scanner sc = new Scanner(System.in);
// Generate random number to guess between <min, max>.
int number = min + (int)((max + 1) * Math.random());
System.out.println(text1A + min + text1B + max + ". " + text2A + attempts + text2B);
for (int i = 1; i <= attempts; i++) {
System.out.println(text3);
// Get the guess from the player.
guess = sc.nextInt();
// If the number is guessed correctly.
if (number == guess) {
System.out.println(text7);
return;
}
// If the guess is smaller than real number.
else if (number > guess && i != attempts) {
System.out.println(text4 + guess);
}
// If the guess is bigger than real number.
else if (number < guess && i != attempts) {
System.out.println(text5 + guess);
}
}
// Player lost. Reveal the right answer.
System.out.println(text6 + number);
}
}
It contains variables for the minimum (min) and maximum (max) value of the guessed number, the number of attempts and the player’s current guess.
The text constants (text1A to text7) contain messages that are printed during the game to inform the player of the guessing status.
createGame() method
This method allows you to set the parameters of the game: the minimum and maximum number and number of attempts the player has.
play() method
This method triggers the game itself.
It generates a random number between the min and max values.
It informs the player of the range of numbers and the number of attempts available.
The player enters a guess and the program checks if this guess is correct.
If the guess is lower or higher than the generated number, the program informs the player and allows another attempt.
If the player guesses the correct number, the game ends with a successful outcome.
If the player does not guess even after the last attempt, the program reports the correct number.
Main Class
import games.NumberGuessing;
public class Main {
public static void main(String[] args) {
NumberGuessing game = new NumberGuessing();
game.createGame(1, 100, 5);
game.play();
}
}
Starts the game by creating a NumberGuessing object and calling the createGame() and play() methods.
The game is set up with numbers from 1 to 100 and the player has 5 tries to guess the number.
The Java game process:
The program prints out a range of numbers and informs the player of the number of attempts.
The player enters their guesses and the program gives them feedback on whether to guess higher or lower.
If they guesses correctly, the game ends in success, otherwise the player loses and the program prints out the correct number.
The program works as a simple game to train guessing and logical thinking.
The output of the game can look like the following:
Here you can download the source code of the programmed Java NumberGuessingGame.
In this article, we have demonstrated programming games for Java beginners. We hope that how to program a Java game wasn’t as difficult as you might have expected and that you’ll be eager to keep improving your programming skills.
If you’re a Java developer looking for a job, check out our employee benefits and respond to our job offers!