Data structures: characteristic and types
In today’s digital world, data has become one of the most valuable commodities, whether it is social networks that analyse billions of interactions between communicating people every day in the virtual space of the Internet, medical systems that store information about patients and diseases who have come to the doctor for an examination, or digital trading instruments such as Bitcoin, which is now hitting the magical level of one hundred thousand dollars per Bitcoin.
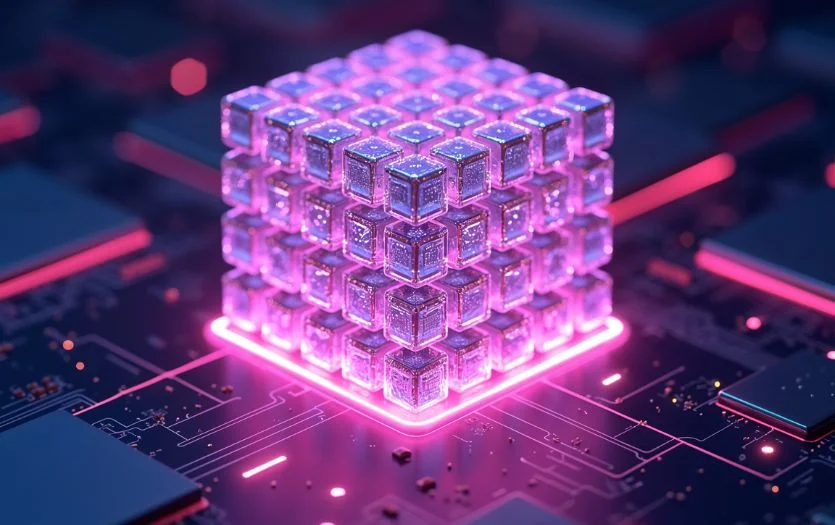
V článku sa dozvieš:
Data is ubiquitous and is becoming a driving force for innovation in all areas of human activity. But using it effectively requires an understanding of its structure and processing – which brings us to data structures. Data itself would be useless to us if we did not know how to organise, store and process it effectively.
Imagine a library without any system for arranging books – a chaos in which it is almost impossible to find what we are looking for. Similarly, the world of computer systems could not cope with the current volume of data, without well thought out and optimised data structures.
These structures, which are an invisible architecture for us users, are responsible for the correct categorization of data, storage and processing in such a way that, for example, a secretary working with office software, a player of the latest computer game, a customer shopping during Black Friday in an online store, or a query programmer in a large database, bring almost instantly exactly the data that they need or expect at that moment in time.
In this article, we delve into the fascinating world of data structures. We’ll talk about the basic ones like arrays and lists. From the basic ones, like arrays and lists, to the advanced ones, like hash tables or binary trees, we’ll show how each plays a key role in solving a variety of computational problems. You’ll learn why some structures are ideal for fast searches, others for storing large amounts of data, and still others for dynamic data management.
Whether you’re a budding programmer, a curious computer science student looking to better understand the fundamentals of your future craft, or already working in the IT field, this article will give you a practical insight into the importance of choosing the right data structures.
By not understanding the key features, strengths and weaknesses of a given data structure and using them inappropriately and inefficiently, we not only waste valuable data processing time, but also waste hardware resources. As we all know, time is money and, for example, a slow response when searching for goods on an e-shop (by this we mean processing and filtering data according to the user’s criteria) has already discouraged more than one customer from making a purchase.
Data isn’t just meaningless binary numbers or text made up of weird ASCII characters – it’s the foundation of our lives. The world of modern technology is powered by data, efficient data structures and algorithms, and this article will introduce you to some of them.
What is data?
Data are the building blocks of information. They are ordinary facts, numbers, texts, symbols, sounds or images that have no concrete meaning without context. We can think of them as individual pieces of a puzzle – individually they are only small parts of a whole, but when arranged correctly, they can form a beautiful picture or become the solid foundation of something bigger.
Characteristics of the data
- Roughness: data are uninterpreted. For example, the number “42” doesn’t say much on its own – it could be an age, the number of pages in a book, or just a random number.
- Potential for information: when data is processed, analysed or put into context, it is transformed into information. For example, “42 °C” in the context of weather indicates extreme heat.
- Digital notation: in modern computer science, data is represented digitally – in the form of binary values (0 and 1), which are the basis of computer operations.
Categories of data
The data can be structured as follows:
- Structured data – data stored in an organized form, such as tables or databases, where individual values are assigned to specific attributes.
- Unstructured data – text documents, images, videos or audio recordings that do not have a predefined structure.
- Semi-structured data – data that has some organizational structure, such as XML or JSON files, but is not as strictly structured as tables.
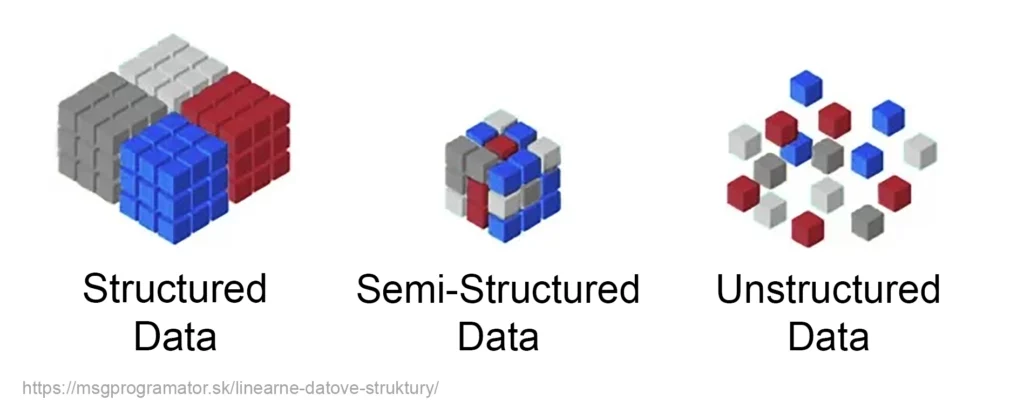
The meaning of data
Data forms the basis of all decisions in the modern world. Companies use it for market analysis, scientists use it for research, doctors use it for diagnosis and treatment, and governments use it for budget planning. Artificial Intelligence (AI) as we know it today would never have come about without it. Thanks to technologies that enable the collection, processing and analysis of vast amounts of data, we are now able to uncover patterns, make predictions and use statistics to find new knowledge that would otherwise remain hidden.
Data structures
Data structures represent a way of organizing, storing, and processing data so that it can be used efficiently in computer programs. They give data a particular form and determine how the data will be arranged, but also how it can be manipulated. They are the basic building blocks of a program.
Design principles for data structures
We always try to optimize the speed of operations such as data search, retrieval and writing when designing a data structure or selecting an existing one. A secondary thing to keep in mind is that efficient data structures should minimize memory footprint. This includes not only the space for storing data, but also auxiliary structures, indexes or metadata that help in faster retrieval of the currently requested information. The implementation of the data structure should be as simple as possible for the data in question to eliminate the risk of errors. It is also essential to design the data structure to be flexible and easily extensible for different applications.
Efficient selection and implementation of data structures has a major impact on the complexity of the algorithms that use them and thus the overall performance of the application.
Types of data structures
Data structures can be divided according to their data storage architecture into:
- Linear Data Structures
In a linear data structure, all elements are arranged in a linear or sequential order. Typical examples of this type of structure are:array,linked list, stack,queue. - Nonlinear Data Structures
In a nonlinear data structure, elements are arranged hierarchically or in a multilevel complex data structure. Typical examples of this type of structure are:tree andgraph. - Tabular data structures
This includes data structures that operate onhash table principles.
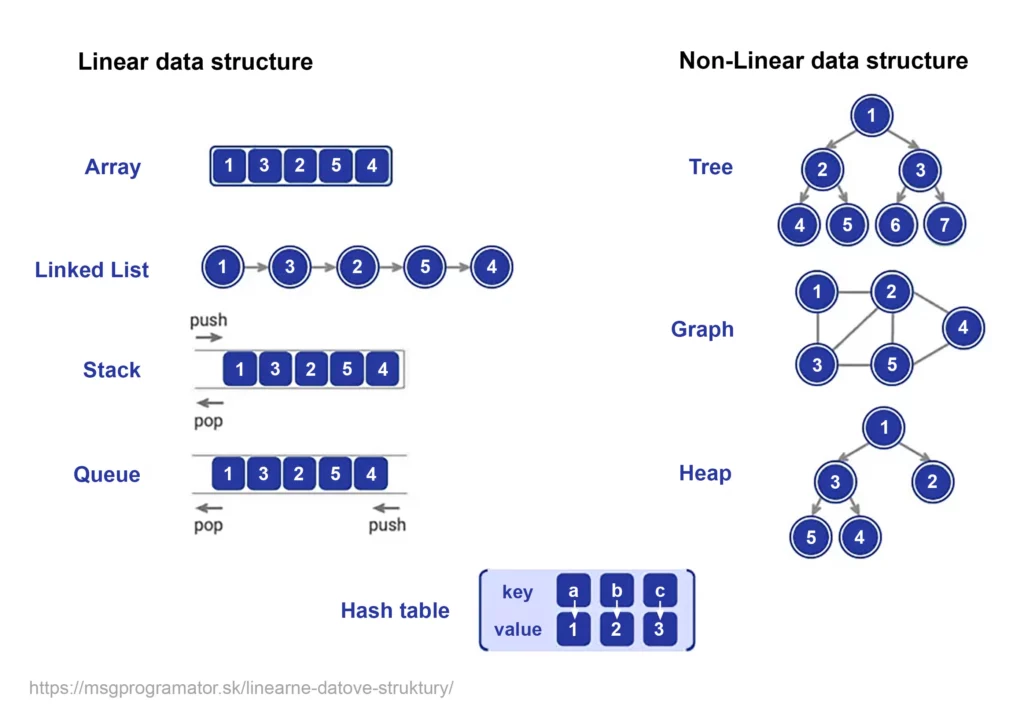
Basic operations with data structures
Search – any element in the data structure can be searched.
Insert – inserting a new element into the data structure.
Update – replacing an element with another element in the data structure.
Delete – removing an existing element from the data structure.
Sort – elements of the data structure can be sorted either ascending or descending. In our past articles, we have exhaustively covered data sorting and sorting algorithms.
Abstract data type vs. Data structure
A data structure is sometimes mistaken for an abstract data type (ADT).
The ADT says what is to be done, and the data structure says how it is to be done. In other words, we can say that the ADT provides us with the design, while the data structure provides the implementation part. In a particular ADT, different data structures can be implemented. For example, The ADT stack can use an array or linked list data structure at the data level. The decision of what to use is up to the programmer and their specific requirements.
Linear data structures
We will now discuss in detail linear data structures, their basic properties, types, advantages and disadvantages, as well as the most common use cases/examples.
Array (Array)
In programming, arrays are one of the most expensive and widely used data structures. They store elements in a contiguous block of memory, with each element accessible by a numeric index. While arrays offer an access time of O(1), which is excellent for read-intensive operations, the efficiency of write operations (insertion and deletion) can vary greatly depending on the location of the operation in the array and the overall size of the array.
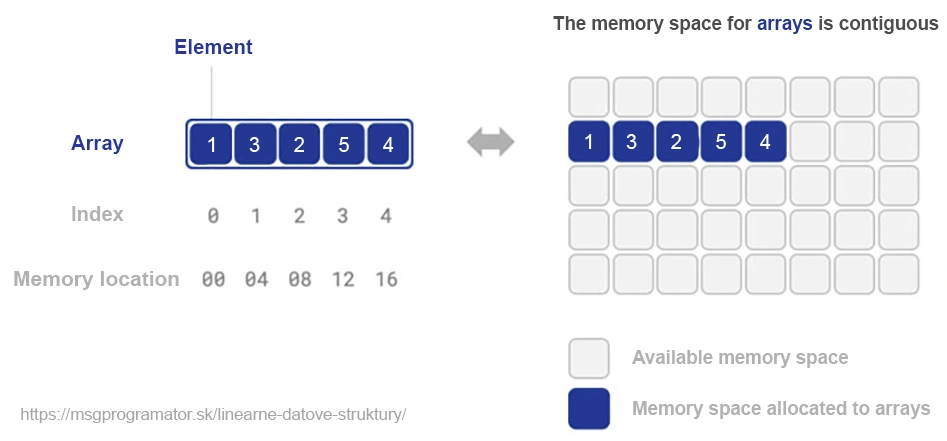
Basic field properties
- Fixed size: once the field is declared, its size is fixed and cannot be changed during the run. Therefore, we need to know in advance how many element arrays need to be created. However, in Java this limitation can be circumvented by using the dynamic ArrayList array structure.
- Homogeneous elements: arrays usually store elements of the same data type, thus ensuring uniformity of the stored data.
- Contiguous memory allocation: Elements are stored in memory locations allocated consecutively, allowing efficient access to elements using indexes.
Field types
- One-Dimensional arrays – a series of elements placed in a row.
- Multidimensional arrays – arrays that contain one or more arrays as their elements. This allows you to create a data representation in multiple dimensions. For example, The 2D array is used for matrices.
Advantages of using the field
- Direct access to the elements: Direct access to any element using its index provides efficient read operations.
- Memory efficiency: it is very efficient for storing a large number of elements in a row. There is no need to allocate extra memory for references to other elements as in a linked list.
Disadvantages of using the field
- Fixed size: the size of the static array is determined at compile time, which makes this structure inflexible.
- Inefficient insertion and deletion: especially for elements at the beginning or in the middle of the array, as this requires scrolling elements.
- Waste of memory: If not fully utilized, it blocks memory unnecessarily.
Use of the field
- Storing data: especially data that has been previously sorted and will not be reordered in the field.
- Lookup tables: arrays can efficiently implement lookup tables that allow quick access to pre-calculated values.
- Implementation of other data structures: arrays are the basic structure for more complex data structures such as heaps, hash tables, and dynamic arrays (e.g., Java Vector).
- Algorithms: They are used in a wide range of algorithms, including sorting and retrieval algorithms, where random access to elements greatly optimizes performance.
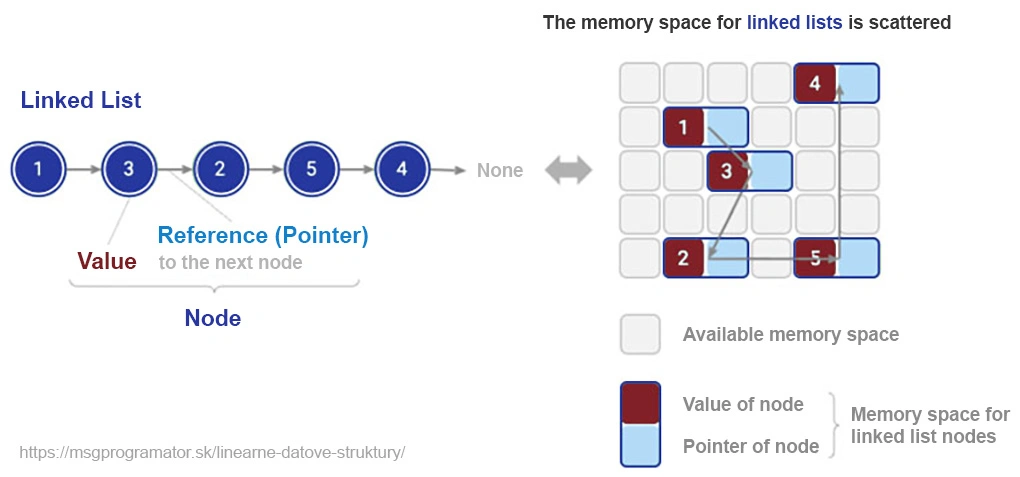
List (Linked List)
A linked list is a basic data structure that is distinguished from an array by its dynamic nature and flexibility. Unlike arrays, which require a contiguous block of memory, a linked list consists of nodes scattered in memory, with each node containing a value and a reference (pointer) to the next node. This feature allows easy manipulation of elements, but at the cost of lower efficiency for direct access.
Basic list properties
- Dynamic size: the size of the list is not fixed and can change dynamically during the program runtime – the list is ideal for applications where the size of the data is not known in advance.
- Distributed memory allocation: List nodes are not stored in contiguous memory blocks, but are linked through links, allowing elements to be added and deleted without the need to rearrange memory.
- Sequential access: unlike arrays, which allow direct access to elements via an index, a list requires sequential traversal from the beginning to the desired node.
Types of lists
- Singly listed list: each node contains a value and a reference to the next node. The last node points to null to indicate the end of the list.
- Circular listed list: the last node points back to the first node, creating a circle – useful for applications that require repeated access to elements.
- Doubly listed list: each node contains a reference to the previous and next node, allowing traversal in both directions.
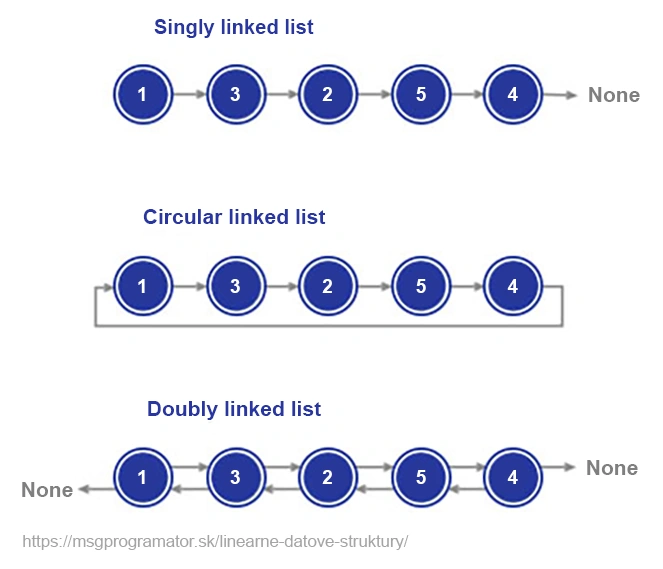
Benefits of using a list
- Efficient manipulation: adding and deleting elements is simple and efficient if we know the reference to the edit location, with O(1) time complexity.
- Flexibility: dynamic sizing eliminates the need to specify capacity up front and avoids memory waste.
- Implementation of complex structures: lists are the basis for other data structures such as stacks, queues and graphs.
Disadvantages of using a list
- Slow access: random access to elements is not possible – each access requires traversal from the beginning of the list, which has a time complexity of O(n).
- Memory consumption: each node requires additional memory to store references, which can be inefficient with a large number of small elements.
- A more complex report: Pointer manipulation when adding or deleting nodes can be error-prone.
Using the list
- Dynamic data structures: lists are used where data is frequently added or removed, for example in tasks where the number of elements is constantly changing.
- Representation of mathematical structures: used to represent polynomials or other dynamic data sets.
- Implementing stacks and queues: many data structures are built on linked lists.
- Garbage collection: memory management algorithms, such as mark-and-sweep, use lists to keep track of blocks of memory.
Examples of use
Imagine managing your history in a browser. Each visited site is stored as a node in a list. When you go back to the previous page, you simply move to the previous node, an ideal example where the list excels. Lists are indispensable for tasks requiring flexibility and dynamic data operations, despite not being suitable for all types of tasks.
Stack
The stack is one of the basic data structures that works on the principle of “Last In, First Out” (LIFO). Think of it as a set of plates stacked on top of each other – we always add a new plate on top and always reach for the top one when removing it. This simple but extremely useful structure has a wide range of applications in programming and computer science.
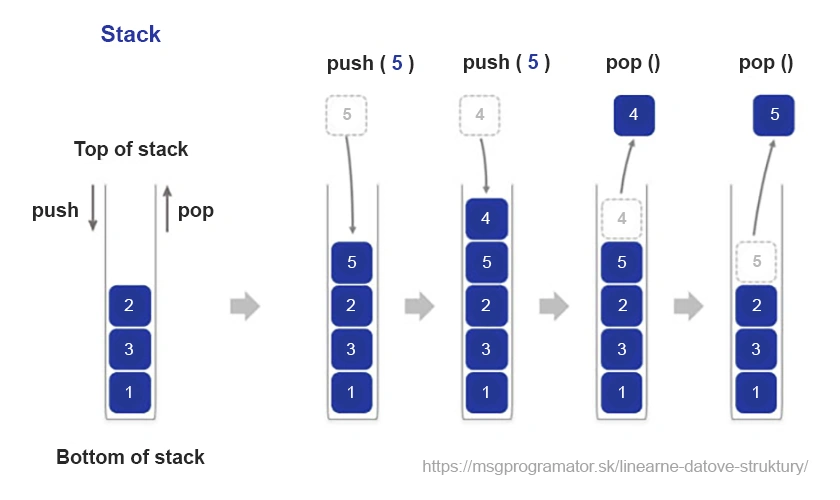
Basic features of the tray
- Top-only operations: all operations (adding and removing an element) are performed at the top of the stack, thus maintaining its LIFO nature.
- Dynamic size: depending on the implementation (e.g. using a list), the stack can dynamically grow or shrink as the program runs.
- Sequential access: unlike arrays, the stack does not have random access – we can only work directly with the last inserted element.
Key stack operations
- Push: Adds an element to the top of the stack.
Pop: Removes and returns an element from the top of the stack.
Peek (Top): Displays the element at the top of the stack without removing it.
IsEmpty: Checks if the stack is empty.
Advantages of using a tray
- Speed of operations: the operations of adding and removing elements are very efficient, with a time complexity of O(1).
- Ease of implementation: the stack is simple to understand and implement, whether using an array or a list.
- Specific Use: The tray is ideal for solving problems where LIFO-style processing order needs to be maintained.
Disadvantages of using a tray
- Restricted access: access is only possible to the element at the top, making it unsuitable for tasks requiring direct access.
- Memory dependency: in the case of an array implementation, the stack may require memory reallocation if its capacity exceeds its original size.
Tray use
- Managing function calls: stacks are used to manage function calls in programs. Each time a function is called, its context is stored on the stack, and restored when the function is finished.
- Syntax check: when verifying the correct enclosure of parentheses or other paired symbols in code, the stack ensures that the order is correct.
- Backtracking algorithms: used for tasks such as solving mazes or Sudoku where you need to return to a previous decision point.
- Implementation of undo functions: in applications where we want to undo the last action, the stack keeps a history of the actions performed.
Example of use
Imagine a text editor with a back function. Every change we make is added to the stack. When we click “undo”, the last change is removed and our document returns to its previous state.
While stacks are specific in their simplicity and limitations, these features make them indispensable in many applications and algorithms where the order of execution of operations must be precisely preserved. For more information, read the Java Stack article .
Front (Queue)
A queue is a basic data structure that operates on a first in, first out (FIFO) basis. It can be thought of as a queue of orders waiting to be processed – the first one to arrive will also be the first to be processed. This structure is extremely useful in situations where it is important to maintain the order of processing.
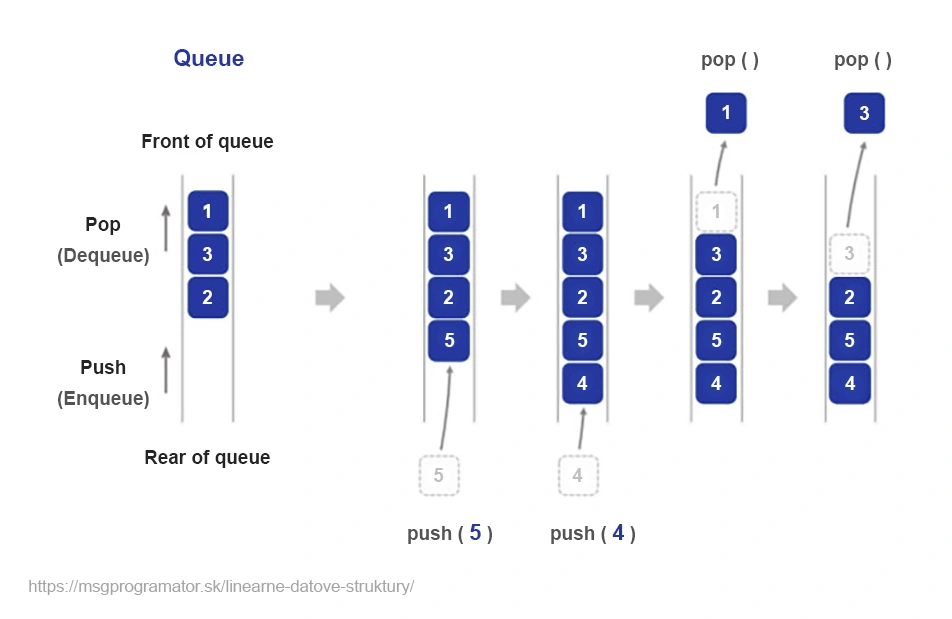
Basic queue properties
- End operations: new elements are added to the end (enqueue) and removed from the front (dequeue), thus preserving FIFO behavior.
- Dynamic size: depending on the implementation, the queue can grow or shrink as the program runs.
- Sequential access: access is possible only to the element at the beginning (front) or at the end (rear) of the queue.
Key operations of the front
- Enqueue: Adds an element to the end of the queue.
Dequeue: Removes and returns an element from the front of the queue.
Peek (Front): Displays the first element in the queue without removing it.
IsEmpty: Checks if the queue is empty.
Front types
- Simple Queue: classic FIFO structure where elements are added to the end and removed from the beginning.
- Circular Queue: a more efficient variant that reuses the freed space by linking the last index back to the first.
- Priority Queue: each element is assigned a priority and is processed according to that priority, regardless of the order in which it is added.
- Double-Ended Queue (Deque): allows adding and removing elements at both ends.
Advantages of using queues
- Order Preservation: ideal for applications where it is important to process elements in the order of their arrival.
- Efficiency: adding and removing elements is time-efficient, with time complexity O(1) if implemented correctly (e.g., a circular queue).
- Simplicity: the queue concept is simple to understand and implement.
Disadvantages of using queues
- Restricted access: only the first and last elements can be manipulated, making it unsuitable for tasks requiring random access.
- Memory inefficiency: when implemented using arrays, a simple queue can be inefficient if the freed space is not recycled.
Using queues
- Process management: operating systems use queues to schedule processes, where tasks wait to be executed in the order they arrive.
- Data stream processing: in network communication and video streaming, queues are used to control the reception and processing of data.
- Breadth-First Search: In algorithms such as Breadth-First Search (BFS), queues provide a sequential search of adjacent vertices in the graph.
- Request Handling: Web servers or databases use queues to process incoming requests (HTTP requests) in the order in which they are received.
Example of use
Imagine a supermarket checkout. Every customer who comes in is placed at the end of the queue (enqueue). The cashier always serves the customer at the front of the queue (dequeue). This process preserves the order of arrival of customers.
Queues are useful wherever tasks or data need to be processed efficiently in the order they arrive. Despite the limitations of their sequential approach, they are one of the most important structures in programming.
Nonlinear data structures
We will now discuss in detail non-linear data structures, their basic properties, types, advantages and disadvantages, as well as the most common use cases/examples.
Tree
A tree is a basic nonlinear data structure that organizes data hierarchically. It consists of nodes, where each node has one parent node – the parent (excluding the root node) and may have zero or more child nodes – the children. This structure enables efficient data processing, especially in searching, sorting and representing relationships.
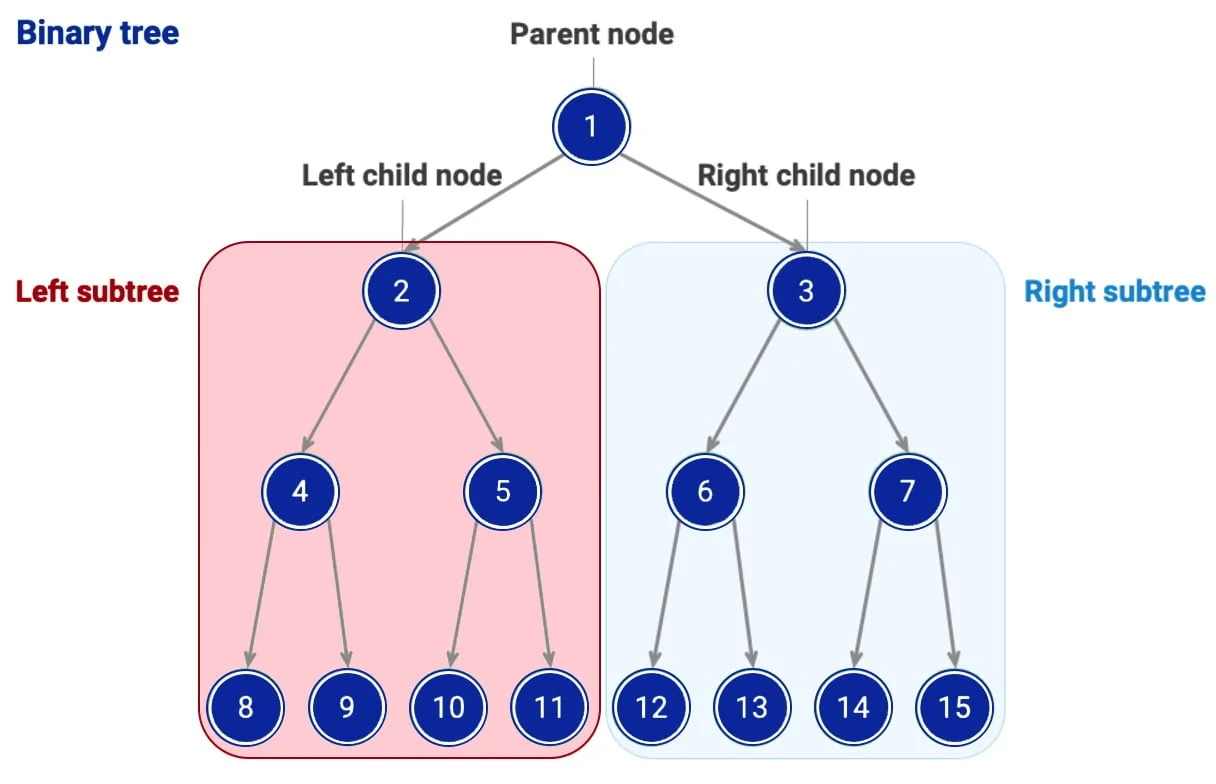
Basic characteristics of the tree
Hierarchical structure: trees are arranged in hierarchical layers, with the root at the top and the nodes below it representing the next levels.
Nodes and edges: each node contains data and links to its child nodes. Edges represent connections between nodes.
Root: It is the highest node of a tree that has no parent node.
Leaves: are nodes that have no children (also known as terminal nodes).
Height of the tree: The number of edges on the longest path from root to leaf.
Types of trees
Binary tree: each node can have at most two descendants – a left and a right node.
Binary Search Tree (BST): a binary tree where the left subtrees contain values less than a node and the right subtrees contain values greater than a node.
Balanced Trees: trees, such as AVL or red-black trees, that maintain a balanced height for more efficient operations.
N-ary tree: nodes can have up to N child nodes.
Trie: Specialized tree for efficient searching, for example when working with words or prefixes.
Benefits of using a tree
- Efficient searching and sorting: trees, especially binary search trees, allow fast searching and organizing of data.
- Flexibility of representation: they allow to model hierarchical relationships, such as organizational structures, file systems or taxonomy.
- Dynamic nature: Trees can grow or shrink with no fixed size.
Disadvantages of using a tree
- More complex implementation: working with trees requires managing pointers to tree nodes, which can be error-prone.
- Memory requirement: each node needs additional memory for references to its child nodes.
- Inefficiency for unbalanced trees: trees that are unbalanced may degrade to a linear structure, leading to degraded performance on O(n).
Use of trees
- Database indexes: B-trees and their variants are used to efficiently search and manage large datasets.
- File systems: trees represent the structure of folders and files on a disk.
- Pathfinding in graphs: trees are the basis of algorithms for pathfinding or graph coverage.
- Data compression: Huffman trees are used in compression algorithms such as zip or gzip.
Example of use
Imagine a family tree – the root of the tree represents the oldest ancestor and the nodes below represent its descendants. This hierarchy is a direct example of using a tree to organize and clearly represent data.
Trees are one of the most important data structures in computer science. Their ability to model complex relationships and efficiently manipulate hierarchical data makes them an integral part of modern algorithms and applications.
Graph (Graph)
A graph is a non-linear data structure that represents objects (nodes, called vertices) and the relationships between them (called edges). This structure is extremely versatile and is used to model a variety of situations such as social networks, traffic maps, or relationships between entities in databases.
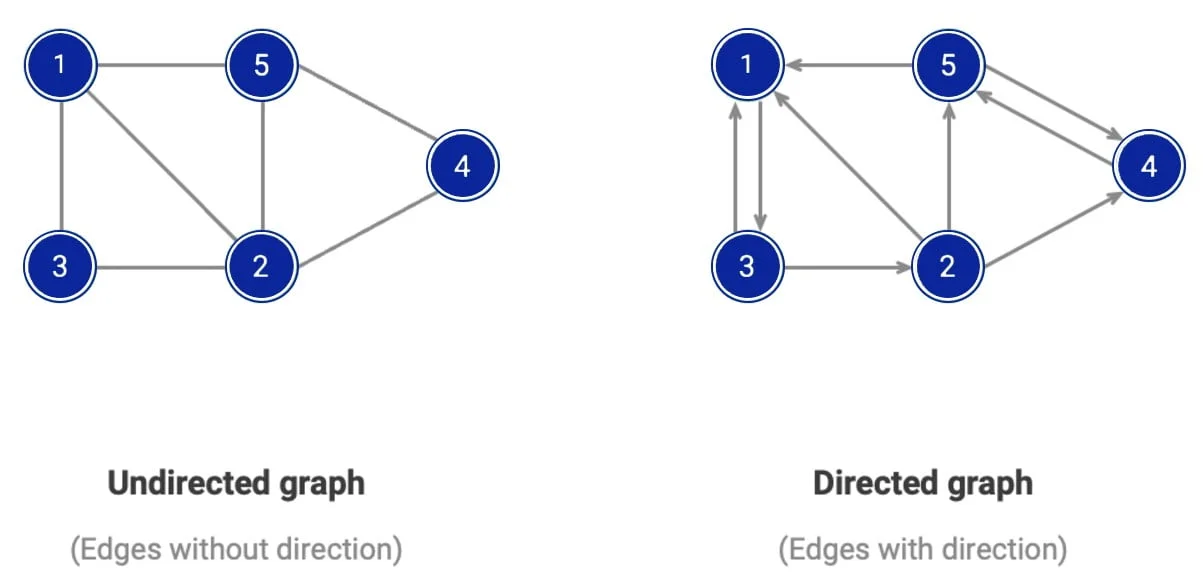
Basic chart properties
Vertices (Nodes): the basic parts of the graph that represent objects or entities.
Edges: connections between vertices that represent relationships or interactions.
Orientation: the graph can be oriented (directed edges, e.g. a one-way street) or non-oriented (no direction, e.g. a two-way road).
Edge weighting: some charts contain weights on the edges to represent values such as distances, costs or time.
Types of graphs
Undirected Graph: edges have no direction, the connection between two vertices is mutual.
Directed Graph or Digraph: Edges have a direction and connect vertices in a specific order.
Weighted Graph: the edges are assigned a value (weight).
Cyclic Graph: contains closed paths (cycles).
Acyclic Graph: contains no cycles.
Tree Graph or Tree: a special case of an acyclic graph where each vertex is accessible via a single path.
Connected Graph: there is a path between every two vertices.
Benefits of using graphs
- Flexibility of representation: graphs are suitable for modelling any relationship, from network topologies to human interactions.
- Efficient search: many algorithms are optimized to work with graphs, such as Dijkstra’s algorithm for finding the shortest path.
- Visualisation option: graphs are intuitive to understand and visually interpret, making data analysis easier.
Disadvantages of using graphs
- Memory consumption: storing large graphs, especially if they are dense, can be very memory intensive.
- Complexity of operations: implementing and managing graphs can be complex, especially with dynamic changes.
- Potential inefficiency: some graph algorithms have high time complexity, which can be problematic for large graphs.
Use of graphs
- Social networks: Modeling relationships between users, such as friendships or followings.
- Navigation and transport: representation of roads, railways or air routes.
- Process optimization: finding the most efficient path, minimum cost tree or maximum flow.
- Web crawlers: search engines use graphs to index and analyse the connections between web pages.
- Biology and chemical structures: representation of molecules, gene networks or evolutionary relationships.
Example of use
Imagine an urban transport network. Each place (stop) is a vertex and the connection between stops is an edge. To find the fastest path between two places, we use an algorithm that traverses the graph and identifies the shortest route.
Graphs are indispensable in solving complex problems where it is crucial to understand and be able to analyse the relationships between objects. Thanks to their versatility and the rich possibilities of their application, they have become the basis of many areas of modern computer science.
Tabular data structures
Now we will discuss in detail the tabular data structures, their basic properties, types, advantages and disadvantages, as well as the most common use cases/examples.
Hash Table
A hash table is a data structure that implements an associative array in which values (data) are stored and retrieved based on a unique key. It uses a hash function that converts the key to an index in the array, thus allowing efficient storage and access to the data.
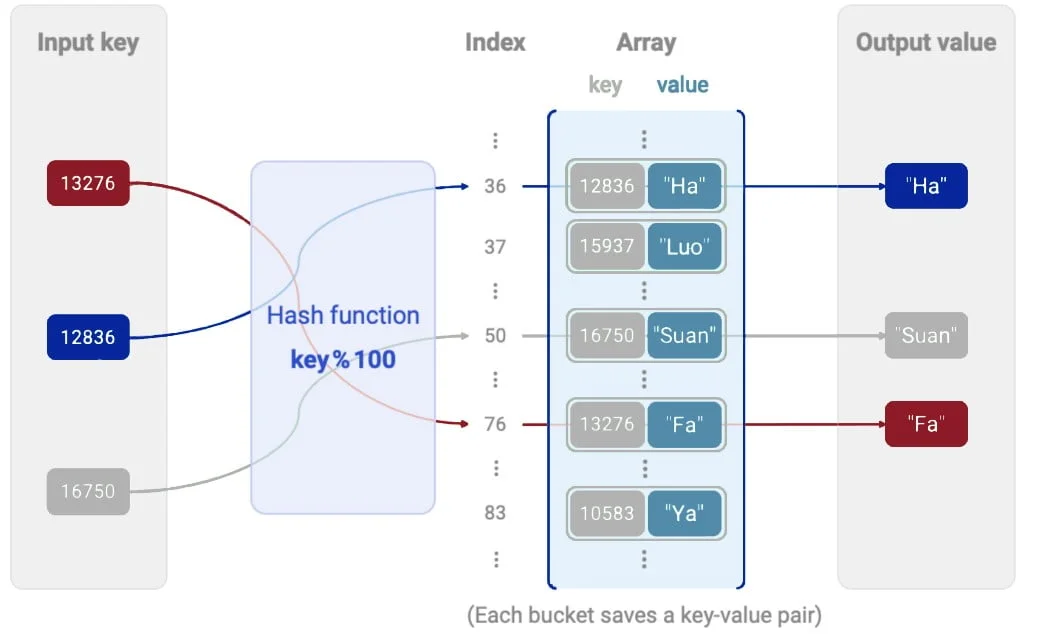
Basic features of the hash table
Hashing function: the key is converted to an index in the corresponding field using this function. The quality of the hash function determines the performance of the table.
Unique keys: each record in the table is assigned a unique key.
Collisions: if two different keys generate the same index, a collision occurs, which is resolved by specific techniques (e.g., separate chaining).
Collision resolution
Separate chaining: each index in the table contains a list or other structure that stores all values with the same index.
Open addressing: if a collision occurs, another free slot is searched for according to a certain pattern (e.g. linear or quadratic probing).
Advantages of using a hash table
- Speed of operations: ideally, search, insert and delete operations have a time complexity of O(1).
- Efficient use of memory: The table dynamically manages space according to the number of elements stored.
- Easy access to data: Enables efficient matching and quick key-based searches.
Disadvantages of using a hash table
- Dependence on hash function: weak function can lead to frequent collisions and reduced performance.
- Memory requirement: with a high number of keys, a large amount of memory may be required.
- Unorganised data: The table does not preserve the order of the records, which can be disadvantageous in some applications.
Using the hash table
- Quick Search: used to implement maps, dictionaries and associative arrays in most programming languages.
- Caching: Tables are the foundation of caches, where fast access to frequently used data is key.
- Duplicate detection: quickly detects if an element already exists, which is useful for filters such as the Bloom filter.
- Database indexes: used to optimize access to data in databases.
- Encryption: hash functions are key in generating unique checksums.
Example of use
Imagine a phone book in which you want to quickly look up a person’s number based on their name. The person’s name serves as a key, which is converted to an index using the hash function. The corresponding phone number is stored on this index.
Hash tables are a powerful and efficient structure for a wide range of applications where fast access to data is a priority. Their ability to efficiently process large amounts of data makes them indispensable throughout the IT field.
Other data structures
I would still like to briefly mention at least some of the lesser known data structures that we may encounter in IT.
Heap
A binary tree that satisfies the following condition (each parent node is larger or smaller than its children). It is used to implement priority queues or in the Heap Sort algorithm, which we have already introduced on our blog.
Trie (Prefix tree)
A trie is a tree table structure optimized for string search, where each node represents a part of a key. It is used in word processors, autocomplete, or database searching.
Disjoint Set
It is used to represent and manage sets with disjunctive elements. It is used in graph algorithms such as the Kruskal algorithm.
Segment Tree (Interval Tree)
A tree designed to efficiently solve problems associated with intervals and ranges of data. It is suitable for quick operations to determine the sum, minimum or maximum of a range.
Fenwick Tree
Efficient structure for updating and searching sums in sequences. It is used in tasks that compute ranges.
K-D Tree (K-Dimensional Tree)
A specialized tree for organizing points in K-dimensional space. It is used in algorithms for image processing, computer vision, and database search.
Suffix Tree
Represents all suffixes of a given string. It is particularly effective in substring search and text-based algorithms.
Matrix Table
Matrix tables are two-dimensional or multi-dimensional arrays that store data in rows and columns. It is used, for example, in linear algebra for working with matrices.
Adjacency Matrix
A two-dimensional matrix where the rows and columns represent the vertices of the graph and the values in the matrix indicate the existence of edges between vertices. It is used to efficiently represent dense graphs and to quickly detect the existence of an edge between two vertices.
Adjacency List
A list where each vertex of the graph contains a list of all its neighboring vertices. Ideal for representing sparse graphs, as it saves memory compared to a neighborhood matrix.
Sparse Matrix
Structure for storing matrices with most zero values, where only non-zero values and their positions are stored. It is used in areas such as machine learning, graph analysis, and modeling large sparse data structures.
Scatter Table
A hash table-like structure that resolves collisions in an alternative way of key dispersion. Efficient for handling large volumes of data with lower risk of clustering collisions in hash structures.
Conclusion
Data structures are an essential part of programming because they provide a way to efficiently organize, process, and manipulate data. Linear data structures (queue, stack, linked list, array) have specific advantages, disadvantages, and areas of application.
Their proper selection and implementation can significantly affect the performance and efficiency of the software. Therefore, understanding the basic principles of data structures is crucial for anyone who wants to delve deeper into programming, algorithms or databases, so that they can use this knowledge to create optimized and innovative solutions.
If you’re looking for a job and you’re a Java developer, check out our employee benefits and respond to our job offers!