SOLID principles of object-oriented programming
Software development is a complex process that requires careful planning, architecture and design. One of the key goals of software development is to ensure that the software is flexible, extensible and sustainable. Enter the SOLID Principles, a set of five core object-oriented programming (OOP) principles that help create high-quality, highly scalable software. These principles were proposed by Robert C. Martin and are known by the acronym SOLID. In this article, we will look at each of these principles in detail.
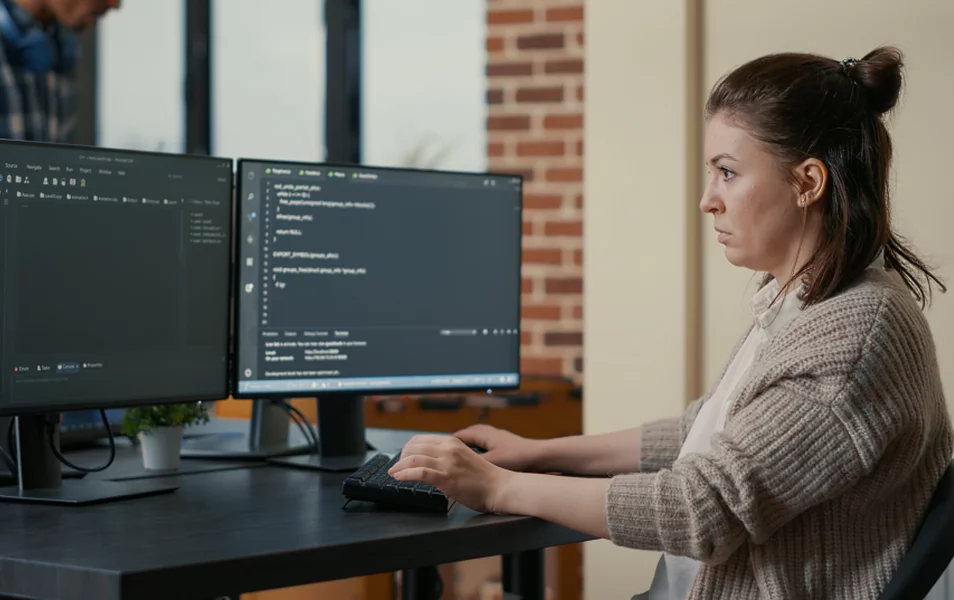
V článku sa dozvieš:
Well-designed software requires a high-quality codebase that is easy to read, understand, maintain (to add/change features, fix bugs) and extend for the future. This saves development time and resources.
SOLID principles of OOP:
S – Single Responsibility Principle
O – Open/Closed Principle
L – Liskov Substitution Principle
I – Interface Segregation Principle
D – Dependency Inversion Principle
Single Responsibility Principle (SRP)
The Single Responsibility Principle states that each module, class or function in a computer program should be responsible for some part of the program’s functionality. They should also encapsulate that part, and their services should be closely related to that responsibility. This ensures that classes are smaller, clearer, easier to understand, and easier to maintain. If a class has multiple responsibilities, it becomes complex and error-prone.
Examples of responsibilities that may need to be separated in software in general:
formatting, parsing, mapping, validation, logging, persistence, notification, error handling, class selection/instance, etc.
Open/Closed Principle (OCP)
The Open/Closed Principle tells us that modules, classes and functions should be both open to extension and closed to modification. This means that we should be able to change the behaviour of a module/class without having to change its source code. We achieve this by using interfaces, abstract classes or inheritance. In this way, we minimize the risk of introducing errors into the existing code when adding new features.
How to use OCP:
Add new functionality by creating new derived classes that inherit from the original base class and allow the client to access the original class through an abstract interface via compositional design patterns, such as the Strategy pattern. Instead of modifying the existing functionality, create new derived classes and leave the original implementation of the class as is. This principle is violated if you ever need to modify the base class.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that an object of a given class should be able to be replaced by an instance of one of its derived classes without changing the behaviour of the program. That is, if we have a class A and a derived class B, we should be able to use B wherever we expect an object of type A. This principle ensures that inheritance and polymorphism work correctly and consistently.
If we can successfully replace an object/instance of the parent class with an object/instance of the child class without affecting the behaviour of the base class instance, we are following the LSP. When this principle is violated, it usually results in a lot of additional conditional logic scattered throughout the application code to check if an object is of a particular type.
As the size of the application grows, duplicate and scattered code becomes a breeding ground for bugs. A very common violation of this principle is partial implementation of interfaces or functionality of the base class, where not all methods or properties are implemented, and instead, we throw an exception (e.g. NotImplementedException).
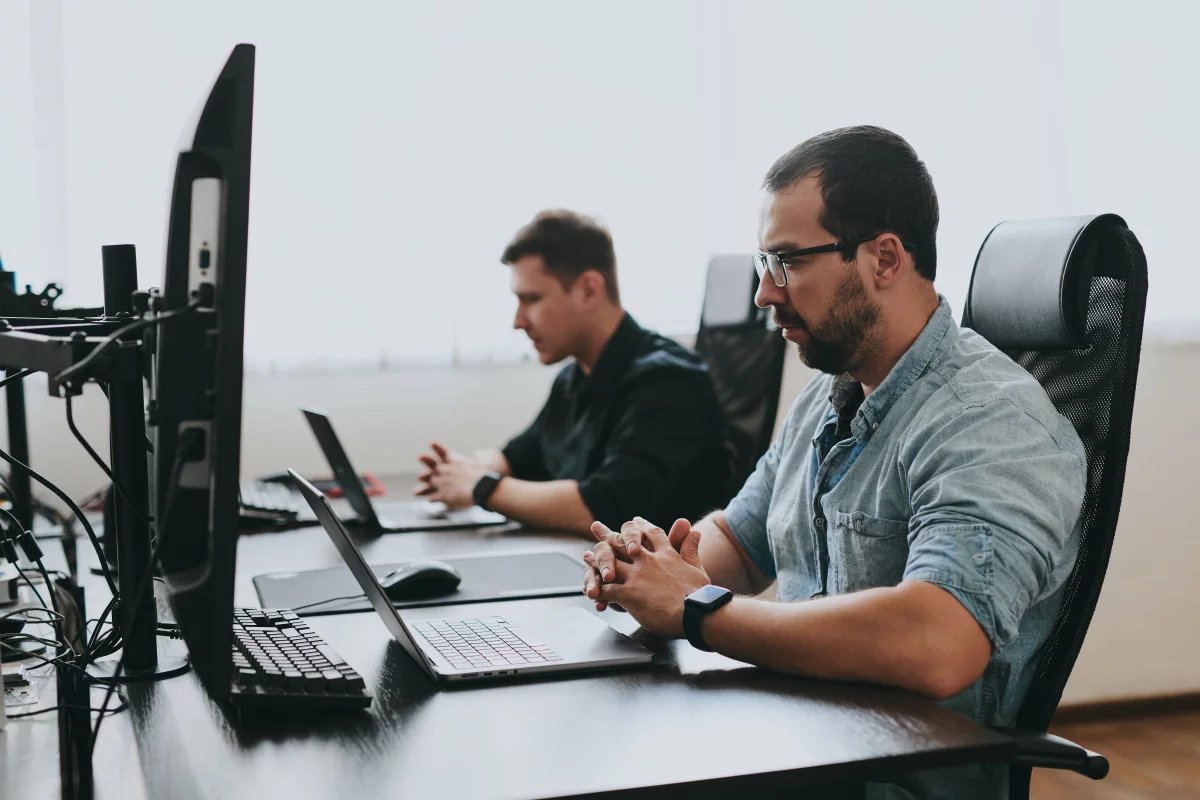
In code that you know will only be used by one client that you can monitor, that’s fine. But in shared code, or even worse, in a framework that is distributed to third parties, such implementations should not occur.
If an interface has more features than you need, use the Interface Segregation Principle (ISP) to create a new interface that contains only the features that your client code needs and that you can fully implement.
Interface Segregation Principle (ISP)
The Interface Segregation Principle tells us that we should create several specific interfaces, rather than one large generic interface. This helps us to minimize dependencies and ensure that classes only implement the methods they need, and are not forced to implement unnecessary methods. Put simply, clients should not be forced to implement methods they don’t use.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that modules/classes at a higher level should not depend on modules/classes at a lower level. Instead, they should both depend on abstractions. Further, abstractions should not depend on implementation details; those details should depend on abstractions.
If one class knows the design and implementation of another, the risk that changes in one class will break the other increases. Always try to keep modules/classes at different levels as little connected as possible. To do this, we need to make both dependent on abstractions rather than knowing each other. This way, the code is flexible and we can easily change it without having to change all the places where the class is used.
Conclusion
Software design principles are recommendations that developers follow when creating software to produce clean and sustainable code. It is a collection of techniques and best practices recommended by many well-known industry experts and authors.
The SOLID principles in programming are an important part of object-oriented software design. Adhering to them helps us produce high-quality, sustainable and easily extensible code.
The Single Responsibility Principle ensures that classes have a single responsibility.
The Open/Closed Principle allows us to change the behaviour of classes without modifying the code.
The Liskov Substitution Principle ensures that derived classes can be used instead of the parent class. The Interface Segregation Principle helps us minimize dependencies between classes.
The Dependency Inversion Principle ensures that we rely on abstractions rather than concrete implementations.
Are you familiar with the Solid principles definition and the Java programming language? If you are looking for a job as a Java developer, see our employee benefits and respond to our latest job offers!