Java design patterns: definition and types
Design patterns definition
A design pattern is a repeatable solution to a commonly occurring software design problem. However, a design pattern is not a finished design that can be directly copied into your code. It is not a specific piece of code but a general concept or template for solving a specific problem, applicable in many different situations. Design patterns can speed up the development process by leveraging tested and proven development practices.
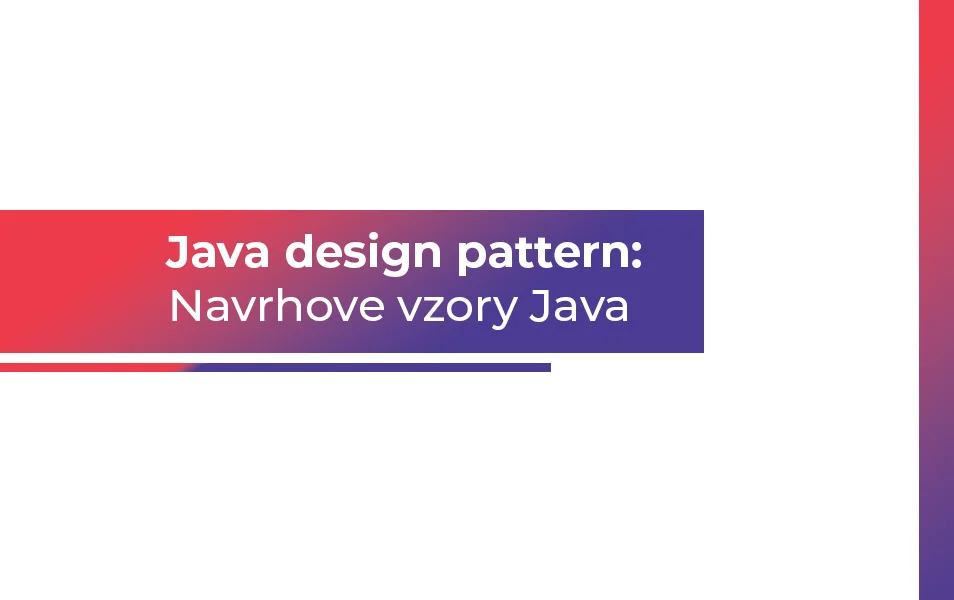
V článku sa dozvieš:
Design patterns categories
Design patterns are categorized into three main types: creational, structural, and behavioural patterns.
Creational patterns
Patterns in this category deal with creating instances from classes.
- Singleton – Ensures that the class has only one instance and provides that as an access point.
- Builder – Allows the construction of a complex object to be broken down into a series of simple steps.
- Prototype – Creates an instance of a new object by cloning an existing one.
- Factory – Provides an interface for creating objects of derived classes.
- Abstract factory – Provides an interface for creating families of related objects without specifying their concrete classes.
Structural patterns
Patterns in this category deal with class structure such as inheritance and composition.
- Adapter – Allows objects with incompatible interfaces to collaborate.
- Bridge – Separates an abstraction from its implementation so that both can vary independently.
- Composite – Allows objects to be composed into tree structures and treated as individual objects.
- Decorator – Dynamically adds or removes responsibilities from objects without affecting others of the same class.
- Facade – Provides a simplified interface to a complex system.
- Flyweight – Allows sharing parts of existing objects to reduce the number of created objects.
- Proxy – Acts as a surrogate to control access to the original object.
Behavioural patterns
Patterns in this category deal with communication between objects.
- Chain of responsibility – Provides a way of passing a request between a chain of objects, where the currently addressed object has the ability to process it or send it on to the next object in the chain.
- Command – Implements the request–response model by wrapping a command and its metadata in an object.
- Iterator – Allows you to navigate through the items in a collection without revealing their base type.
- Mediator – Provides centralized communication between different objects in the system.
- Memento – Allows you to save and subsequently restore the previous state of the object.
- Observer – Provides a mechanism for notifying all interested objects when a change occurs to the observed object.
- State – Allows an object to alter its behaviour when its internal state changes.
- Strategy – Defines a family of interchangeable algorithms, encapsulating each in a separate class.