Java File Handling Part 3: Best practices
Working with files is a core functionality of many Java applications, whether it is reading configuration files, processing user data, or storing application logs. Although Java offers comprehensive file-processing capabilities, effective and reliable file management requires a thorough understanding of best practices and techniques. In this article, we present a number of Java tips and tricks for working with Java files. From handling exceptions and optimizing performance to ensuring file integrity and security, these Java best practices will help you work with files more efficiently. Read other articles in our Java File Handling series:
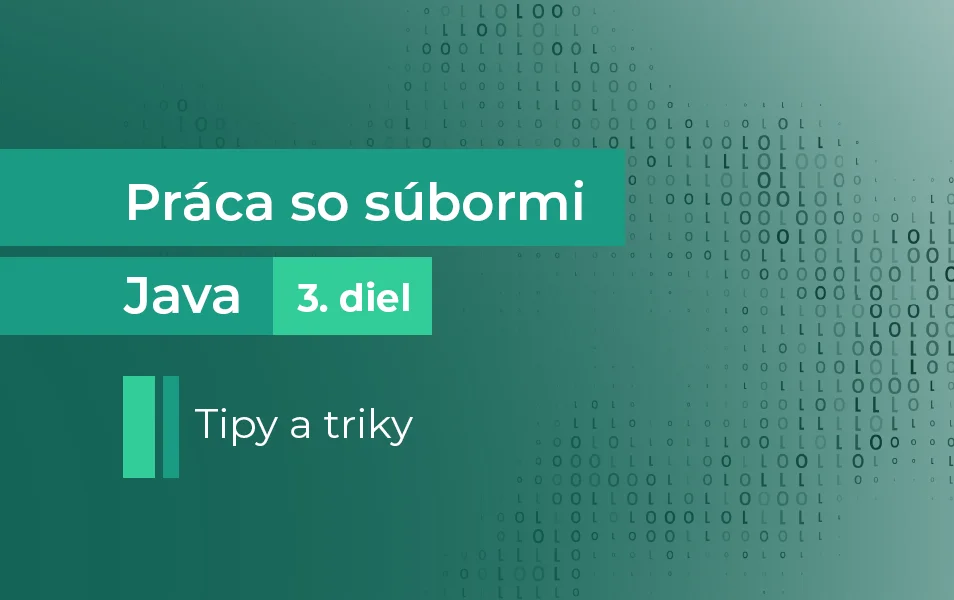
V článku sa dozvieš:
- Part 1: How to Read Java Files Quickly and Efficiently
- Part 2: How to Write Java Files Quickly and Efficiently
Don’t forget to close the file stream
When you finish working with a file, always close the file stream to free up system resources. This can be done using the close() method. For output streams, this method first calls flush() to ensure all buffered data is written before releasing resources.
Learn how to use try-with-resources
When reading or writing to a file, it is important to properly address the opening and closing of the file stream. Many programmers forget about closing, so Java 7 introduced the try-with-resources construct, which automatically closes a stream of files after a try block of code is executed.
Prefer buffered streams whenever possible
Reading or writing large files can hurt performance. The use of buffered streams can help improve performance by reducing the number of I/O operations, so more data is read/written from the buffer and less frequently.
Handle exceptions properly
When reading or writing to a file, it is important to address any exceptions that may occur. Common exceptions include FileNotFoundException and IOException. For example, if we try to read from a file that does not exist, the program throws a FileNotFoundException exception. On the other hand, if we try to write data to a file that does not exist, the file is created first and the program does not throw any exceptions.
Use appropriate encoding
When reading or writing text files, it is important to use the appropriate encoding. The default encoding used in Java is usually UTF-8, but this may not be appropriate for all scenarios.
Use relative file paths
When working with files, prefer relative paths over absolute ones for better portability and easier maintenance.
Manage file permissions
Before performing any file operations, make sure that the appropriate file permissions are set. Wrong permissions can lead to errors or security vulnerabilities. We can use the File.setReadable(), File.setWritable() and File.setExecutable() methods to change permissions.
Use descriptive file naming
When creating new files, it is important to use descriptive file names that are easy to understand and maintain. This can help avoid naming conflicts and make files easier to find and manage.
Use constants for file paths
When working with file paths, it is best to use constants rather than hard-coding paths in the code. This makes it easier to update file paths in the future.
Verify file existence
Before reading or writing to a file, it is important to check that the file exists. This can be done using the File.exists() method.
Use file tracking for real-time updates
If you need to track changes to a file in real time, consider using the Watch Service interface introduced in Java 7. This allows the application to receive notifications when files or directories in the watched folder are modified.
Use file metadata
Extracting metadata such as file size, creation date, last modified date and file type can provide valuable information about the files we are working with. Java has the java.nio.file.attribute package, which provides classes such as BasicFileAttributes for efficiently retrieving such metadata.
Verify file integrity with checksums
To check the integrity of the file, we can calculate its checksum using algorithms such as MD5 or SHA. By comparing the calculated checksum with a known value, we can find out whose file was not copied (or downloaded) during copying (or downloading), or was corrupted or modified during storage.
Delete files safely
When deleting files, always double-check that the file deletion was successful. If deleting a file fails, deal with the situation thoroughly and consider logging the error for further analysis. We can use the File.delete() method to delete files in Java.
Lock files
When working with files, it is important to manage file locking to prevent multiple processes from accessing the same file at the same time. Java provides a number of methods for locking files, including the FileChannel.lock() method.
If you’re a Java developer looking for a job, check out our employee benefits and respond to our job offers!