Java design pattern Command
Today we will look at another Java design pattern from the category of behavioural patterns – Command. Design patterns in this category deal with the interaction between objects and their responsibilities.
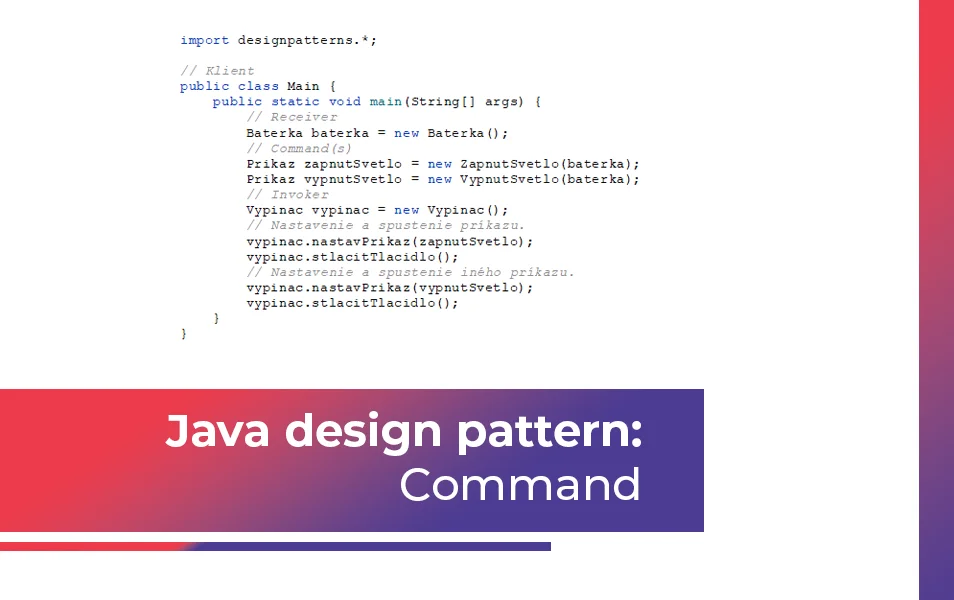
V článku sa dozvieš:
What is the Command design pattern?
The Command design pattern is a pattern that wraps requirements or operations, along with the information to perform them, into separate objects. This information most often includes the method name of the corresponding object along with the method parameters. There are always concepts associated with a pattern: client, command, receiver, invoker. The client decides which order to execute and when. To execute the command, it moves the command object of the invoker object. The invoker does not know anything about a particular object, only its interface. Its job is to execute the command by calling the execute method and, if necessary, store the details of the invoked operation. The receiver then executes the job from the command object.
What problem does the Command design pattern solve?
It solves the problem of separating the object that invokes an operation (the invoker) from the object that performs that operation (the receiver), with the main goal being to provide a flexible connection between the objects that trigger requests and those that process them. These objects contain all the information needed to perform a given operation in the background, and in this way it is possible to work with client requests with different parameters as needed. Requests can be queued for processing, logged or even reverse operations can be implemented.
Example of a Command implementation in Java
The design pattern is a bit more complicated to understand, so I have prepared an example where we will explain the roles of Client, Command, Receiver, Invoker in more detail. We are going to write a simple program that controls a torch using commands.
Command.java
package designpatterns;
// Command rozhranie
public interface Prikaz {
void vykonat();
}
The Command interface defines the execute() operation that all concrete commands will implement.
TurnOnLight.java
package designpatterns;
// Konkrétny príkaz
public class ZapnutSvetlo implements Prikaz {
private Baterka baterka;
public ZapnutSvetlo(Baterka baterka) {
this.baterka = baterka;
}
@Override
public void vykonat() {
baterka.zapnut();
}
}
TurnOffLight.java
package designpatterns;
// Konkrétny príkaz
public class VypnutSvetlo implements Prikaz {
private Baterka baterka;
public VypnutSvetlo(Baterka baterka) {
this.baterka = baterka;
}
@Override
public void vykonat() {
baterka.vypnut();
}
}
Specifically, the TurnOnLight and TurnOffLight commands implement the Command interface and contain a reference to a specific Torch object that will perform the corresponding operation.
Torch.java
package designpatterns;
// Receiver
public class Baterka {
public void zapnut() {
System.out.println("Baterka svieti");
}
public void vypnut() {
System.out.println("Baterka je vypnuta");
}
}
The Torch class represents a receiver and contains the methods that are called when the commands are executed.
Switch.java
package designpatterns;
// Invoker
public class Vypinac {
private Prikaz prikaz;
public void nastavPrikaz(Prikaz prikaz) {
this.prikaz = prikaz;
}
public void stlacitTlacidlo() {
prikaz.vykonat();
}
}
The Switch class represents an invoker and contains a reference to an object of type Command and has a method pressButton() that calls the execute() operation of the corresponding command.
Main.java
import designpatterns.*;
// Klient
public class Main {
public static void main(String[] args) {
// Receiver
Baterka baterka = new Baterka();
// Command(s)
Prikaz zapnutSvetlo = new ZapnutSvetlo(baterka);
Prikaz vypnutSvetlo = new VypnutSvetlo(baterka);
// Invoker
Vypinac vypinac = new Vypinac();
// Nastavenie a spustenie príkazu.
vypinac.nastavPrikaz(zapnutSvetlo);
vypinac.stlacitTlacidlo();
// Nastavenie a spustenie iného príkazu.
vypinac.nastavPrikaz(vypnutSvetlo);
vypinac.stlacitTlacidlo();
}
}
The Main class represents the client. In the Main method we create commands to control the torch and link them to the receiver that will process them. We will also create an invoker object – a switch through which the client sets a specific command that will be executed after the pressButton() method is called. In simple terms, the client sends commands to the switch that controls the torch.
The output from this example is:
Conclusion
The Command design pattern is often used when we need to get rid of the dependency of a requirement on a direct concrete implementation. It has a wide range of applications in GUI, network communication, database transactions, etc.
We have prepared the files with the above example in the form of code that you can run directly in Java. Download the Java Command code here.
If you’re a Java developer looking for work, check out our employee benefits and respond to our job offers!